MongoDB aggregation pipelines explained
MongoDB’s aggregation framework is a robust tool for processing and analyzing data directly within the database. Instead of retrieving data to manipulate it in your application code, you can run complex queries and transformations on the server side. The core of this framework is the aggregation pipeline, which allows you to chain together various stages to perform tasks like filtering, grouping, sorting, and reshaping your data.
How aggregation pipelines work
Aggregation pipelines function by passing documents through a sequence of stages, each performing a specific operation. Each stage receives input, processes it, and passes the output to the next stage. This structure allows you to build complex queries step by step, making it easier to understand and manage the logic of your data transformations.
The aggregation pipeline syntax in MongoDB might look like this:
db.collection.aggregate([
{ aggregationQuery },
{ aggregationQuery },
// Additional stages can be added here
])
Each { aggregationQuery }
represents a different stage in the pipeline. These stages work together to transform and analyze your data effectively. Let’s explore some of the most commonly used aggregation stages in detail.
Filtering data
The $match
stage is used to filter documents based on specific criteria. It works similarly to the find
query but within the pipeline. When you apply $match
, only documents that meet the specified conditions continue to the next stage. This is often one of the first stages in a pipeline, as it helps reduce the number of documents that need to be processed by subsequent stages, improving performance.
For example, if you want to focus on orders for a specific product, you would use:
{ $match: { product: "Laptop" } }
This filters the documents to only those where the product
field equals Laptop.
Joining collections
The $lookup
stage performs a left outer join on two collections. This means it returns all documents from the local collection and, where available, matches them with documents from the foreign collection based on specified fields. This stage allows you to combine related data from different collections into a single result set, which is especially useful when dealing with normalized data.
For example, if you have an orders
collection and a customers
collection, you can use $lookup
to combine them:
{
$lookup: {
from: "customers",
localField: "customerId",
foreignField: "_id",
as: "customerDetails"
}
}
from
: this specifies that we are joining theorders
collection with thecustomers
collection.localField
: thecustomerId
in theorders
collection is used to match with the_id
field in thecustomers
collection.foreignField
: this is the corresponding field in thecustomers
collection that will be matched with thecustomerId
fromorders
.as
: the resulting documents will include a new field calledcustomerDetails
, which will contain an array of matching documents from thecustomers
collection.
This operation would produce a result where each document in the orders
collection is enriched with the corresponding customer details.
{
"_id": 1,
"product": "Laptop",
"amount": 1200,
"customerId": 101,
"customerDetails": [
{
"_id": 101,
"name": "John Doe",
"email": "[email protected]"
}
]
}
Grouping data and aggregating results
The $group
stage is one of the most powerful tools in an aggregation pipeline. It groups documents by a specified field (or fields) and can perform a variety of operations on these groups, such as summing, averaging, counting, or even creating arrays of values. The output of $group
is a set of documents, each representing a group.
Here’s a basic structure of the $group
stage:
{
$group: {
_id: <expression>,
<field1>: { <accumulator>: <expression> },
<field2>: { <accumulator>: <expression> },
...
}
}
_id
: This field determines how the documents are grouped. Documents with the same value are grouped together. You can use a single field, a computed value, or even multiple fields.<field1>
: This is the name of the field in the output document. The value of this field is determined by the accumulator operation (e.g.,$sum
,$avg
,$max
,$min
,$push
, etc.) applied to the grouped documents.
For instance, if you want to calculate the total sales for each product, you would use $group
to group by the product
field and then sum the amount
field for each group:
{ $group: { _id: "$product", totalSales: { $sum: "$amount" } } }
_id
: This is the field by which the documents are grouped. Here, each unique product name becomes a group.totalSales
: This is a new field in the output documents, created by summing theamount
field for each group. The dollar sign before amount indicates that amount is a field in the document, not a literal value. It tells MongoDB to use the value of the amount field from each document being processed.
Ordering results
After grouping or any other operation, you might want to sort the results. The $sort
stage allows you to order documents based on the values of specified fields. You can sort in ascending (1
) or descending (-1
) order.
For example, to sort products by total sales in descending order, you would use:
{ $sort: { totalSales: -1 } }
This ensures that the products with the highest sales appear first in your results.
Shaping your output
The $project
stage is used to include, exclude, or reshape fields in the documents that pass through the pipeline. It’s like selecting specific columns in SQL. You can also use $project
to create new fields or transform existing ones.
For example, if you only want to see the customer name and the amount they spent in the final output, you could use:
{ $project: { _id: 0, customer: 1, amount: 1 } }
This configuration excludes the _id
field from the output and includes the customer
and amount
fields.
Conclusion
MongoDB's aggregation pipelines allow you to perform powerful data processing operations within the database. Each stage plays a critical role in transforming your data step by step. This modular approach lets you build and maintain complex queries more easily, leading to more efficient and maintainable data processing workflows. Aggregation pipelines are a key tool for anyone looking to harness the full power of MongoDB.
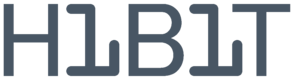
This article is available to HiBit members only.
If you're new to HiBit, create a free account to read this article.
0 Comments